Given an array of characters, compress it in-place.
The length after compression must always be smaller than or equal to the original array.
Every element of the array should be a character (not int) of length 1.
After you are done modifying the input array in-place, return the new length of the array.
Follow up:
Could you solve it using only O(1) extra space?
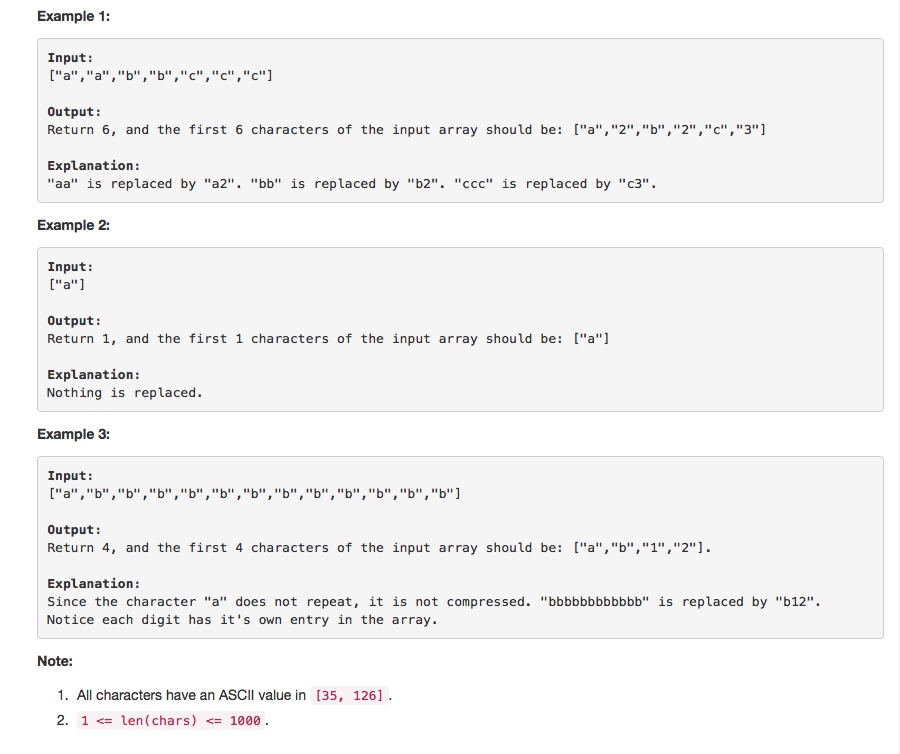
class Solution {
public:
int compress(vector<char>& chars) {
int tol = 1; //统计相同字符的数量
for (int i = 0; i<chars.size(); i++) {
while (i!=chars.size()-1 && chars[i]==chars[i+1]) {
chars.erase(chars.begin()+i+1);
tol++;
}
int log = 0; //标记插入数字是1的情况
int logNum = 0; //统计插入的数字个数
while (log==1||tol!=1 && tol>0) {
chars.insert(chars.begin()+i+1, char(tol%10+'0'));
tol/=10;
log = tol==1?tol:0;
logNum++;
}
i+=logNum;
tol = 1;
}
return chars.size();
}
};