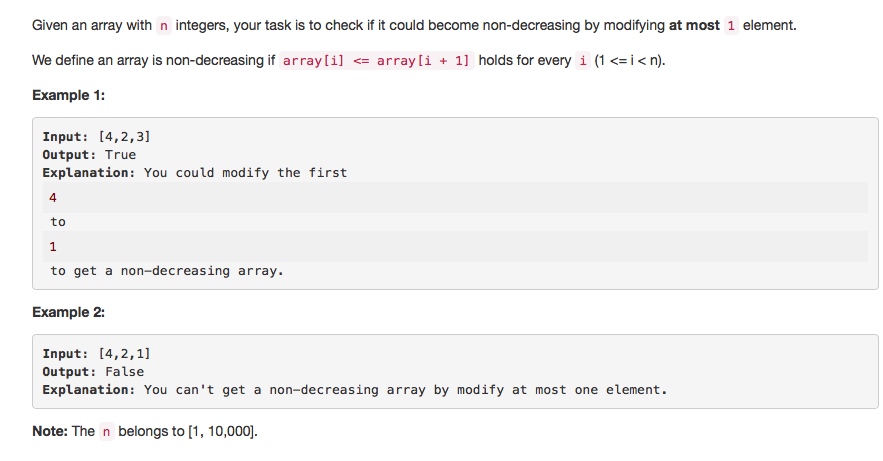
用到了is_sorted
来判断是否升序排列
class Solution {
public:
bool checkPossibility(vector<int>& nums) {
for (int i=0; i < nums.size()-1; i++){
if (nums[i] > nums[i+1]){
int temp = nums[i];
//
// "erase" nums[i], then check if nums is sorted without nums[i]
//
nums[i] = nums[i+1];
if (is_sorted(nums.begin(), nums.end())) { return true; }
//
// "erase" nums[i+1], then check if nums is sorted without nums[i+1]
//
nums[i+1] = nums[i] = temp;
if (is_sorted(nums.begin(), nums.end())) { return true; }
//
// nums is NOT sorted (without nums[i] XOR without nums[i+1])
//
return false;
}
}
return true;
}
};