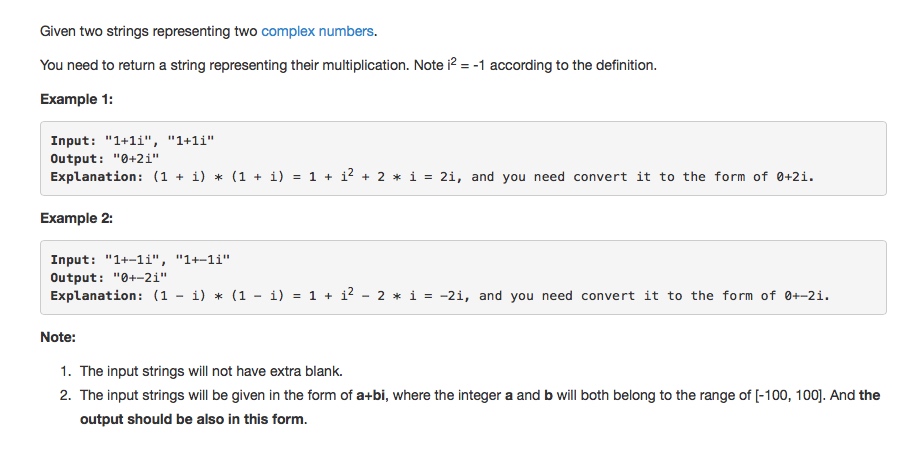
第一次写的复杂版:
string complexNumberMultiply(string a, string b) {
int a1=0 ,b1=0 ,a2=0 ,b2=0;
for (int i = 0; i<a.length(); i++) {
int log = 0;
if (a[i]=='+') {
log = i;
string tmp;
for (int j=0; j<i; j++) {
tmp+=a[j];
}
sscanf(tmp.c_str(), "%d",&a1);
tmp = "";
for (int m = i+1; m<a.length()-1; m++) {
tmp+=a[m];
}
sscanf(tmp.c_str(), "%d",&b1);
}
}
for (int i = 0; i<b.length(); i++) {
int log = 0;
if (b[i]=='+') {
log = i;
string tmp;
for (int j=0; j<i; j++) {
tmp+=b[j];
}
sscanf(tmp.c_str(), "%d",&a2);
tmp = "";
for (int m = i+1; m<b.length()-1; m++) {
tmp+=b[m];
}
sscanf(tmp.c_str(), "%d",&b2);
}
}
string res = to_string(a1*a2-b1*b2)+"+"+to_string(a1*b2+a2*b1)+"i";
return res;
}
精简版:
class Solution {
public:
string complexNumberMultiply(string a, string b) {
int c,d,e,f;
char ret[100];
sscanf(a.c_str(),"%d+%di",&c,&d);
sscanf(b.c_str(),"%d+%di",&e,&f);
cout<<c<<" "<<d<<" "<<e<<" "<<f<<endl;
sprintf(ret,"%d+%di",(c*e-f*d),(d*e+c*f));
string ans(ret);
return ans;
}
};