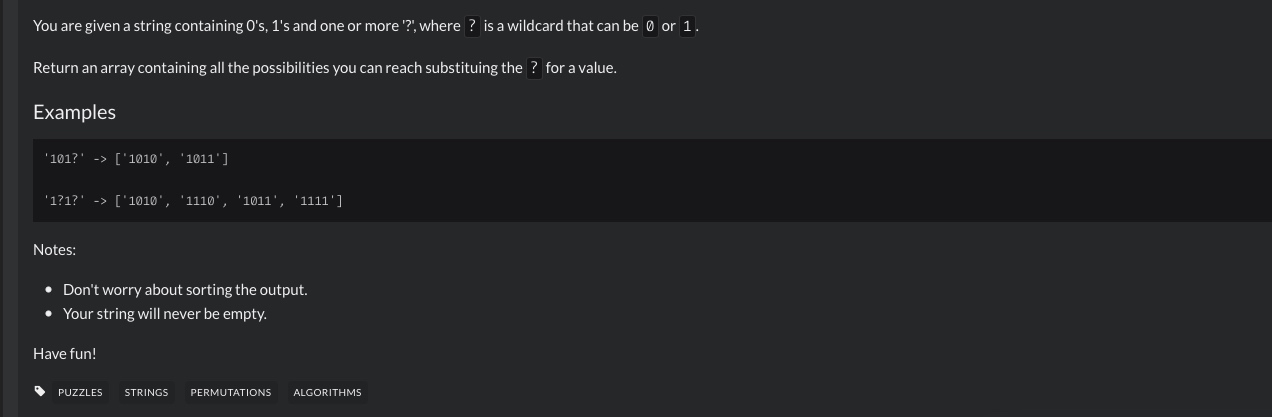
#import <Foundation/Foundation.h>
NSArray *changeTo(NSMutableArray*log, NSMutableArray*res){
if (log.count>0) {
NSRange loc = {[[log lastObject] integerValue],1};
NSMutableArray *cop = res.mutableCopy;
for (NSString *tmp in res) {
[cop addObject:[tmp stringByReplacingCharactersInRange:loc withString:@"0"]];
[cop addObject:[tmp stringByReplacingCharactersInRange:loc withString:@"1"]];
[cop removeObject:tmp];
}
[log removeLastObject];
return changeTo(log, cop);
}else{
return res;
}
}
NSArray *possibilities(NSString *s) {
NSMutableArray *log = @[].mutableCopy;
NSMutableArray *res = @[].mutableCopy;
for (int i = 0; i<s.length; i++) {
if ([s characterAtIndex:i]=='?') {
[log addObject:@(i)];
}
}
[res addObject:s];
NSArray *tol = changeTo(log , res);
return tol;
}
简化版:
#import <Foundation/Foundation.h>
NSArray *possibilities(NSString *s) {
// NSLog(@"User `possibilities` accidentally invoked!\n");
if ([s rangeOfString: @"?"].length == 0) return @[s];
NSString *r = [s stringByReplacingOccurrencesOfString: @"?" withString: @"0" options: 0 range: [s rangeOfString: @"?"]], *t = [s stringByReplacingOccurrencesOfString: @"?" withString: @"1" options: 0 range: [s rangeOfString: @"?"]];
return [possibilities(r) arrayByAddingObjectsFromArray: possibilities(t)];
}