由于AFNetworking
运用了官方NSURLSession
所以其中有一些坑
1.苹果运用NSJSONSerialization解析,出现数字类型精度问题
当服务器给我传回来一个3.0
的数字类型时,在安卓端是没有问题的,但是在iOS这里会出现2.99999
这样的问题。
出现这个问题的原因是:苹果在json解析时,默认为双精度的double类型。
我们相处的解决方案有两种:
1. 跟后台协商将数字型的值改为`字符型`
2. 使用第三方的json解析。
最后我们使用的是第一种方法,因为第二种势必要修改了AFNetwork的源码,开发与维护成本相比较来说要大。
2.json解析失败
由于AF默认的解析方式为0
(返回的对象是不可变的,NSDictionary
或NSArray
):

我们来看一下都有什么选项:

所以当我们服务器返回的json数据是碎片化的(最外层既不是NSArray
也不是NSDictionary
),那么解析的时候就会出错了。
解决方法是:

在0后添加|
字符,增加这种情况,允许碎片化数据。
3. 请求后response的状态码范围问题
正常项目中正常请求成功会返回200,但是服务器若是给你返回了500(我不知道后台为啥会返回这个码),问题就出现了:
这是由于下面的原因:
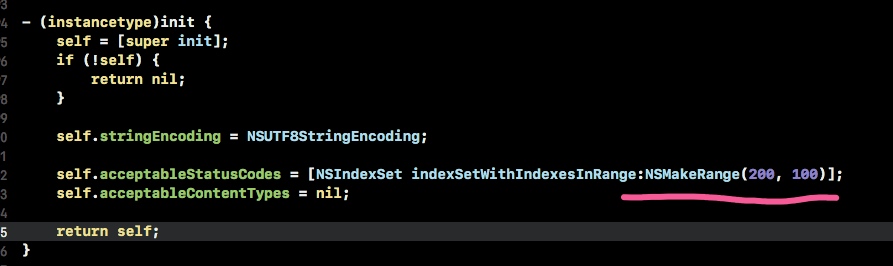
会发现上图中可接受状态码的范围是200-300;
具体解决方法就不说了。。。