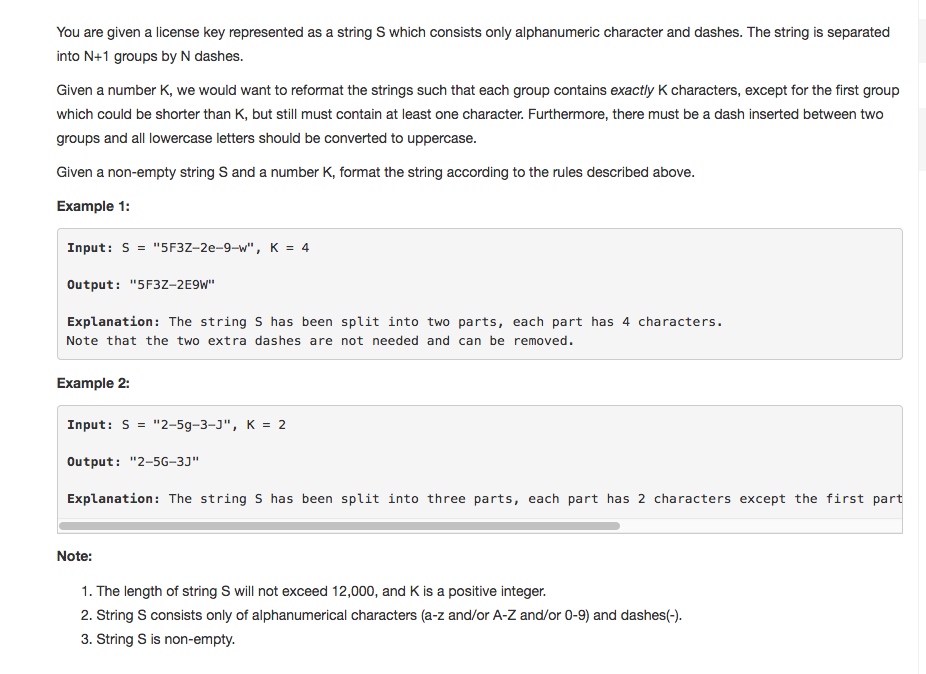
第一版:
int log = 0;
string ele="";
string re="";
for (int i = S.length()-1; i>=0; i--) {
if (S[i]!='-') {
log++;
S[i]=toupper(S[i]);
ele = S[i]+ele;
}
if (log==K||i==0) {
if (i==0) {
re = ele+re;
}else{
re = '-'+ele+re;
}
log = 0;
ele = "";
}
}
return re[0]=='-'?re.substr(1,re.length()-1):re;
第二版:运用栈
class Solution {
public:
string licenseKeyFormatting(string S, int K) {
stack<string> st;
string line;
for(int i=S.length()-1;i>=0;i--){
if(S[i]=='-') continue;
if(line.length()<K){
if(S[i]>='a' && S[i]<='z') line.insert(0, 1, S[i]-'a'+'A');
else line.insert(0, 1, S[i]);
if(line.length()==K){
st.push(line);
line="";
}
}
}
if(st.empty()) return line;
if(line.length() == 0){
line += st.top();
st.pop();
}
while(!st.empty()){
line+='-';
line+=st.top();
st.pop();
}
return line;
}
};