In MATLAB, there is a very useful function called 'reshape', which can reshape a matrix into a new one with different size but keep its original data.
You're given a matrix represented by a two-dimensional array, and two positive integers r and c representing the row number and column number of the wanted reshaped matrix, respectively.
The reshaped matrix need to be filled with all the elements of the original matrix in the same row-traversing order as they were.
If the 'reshape' operation with given parameters is possible and legal, output the new reshaped matrix; Otherwise, output the original matrix.
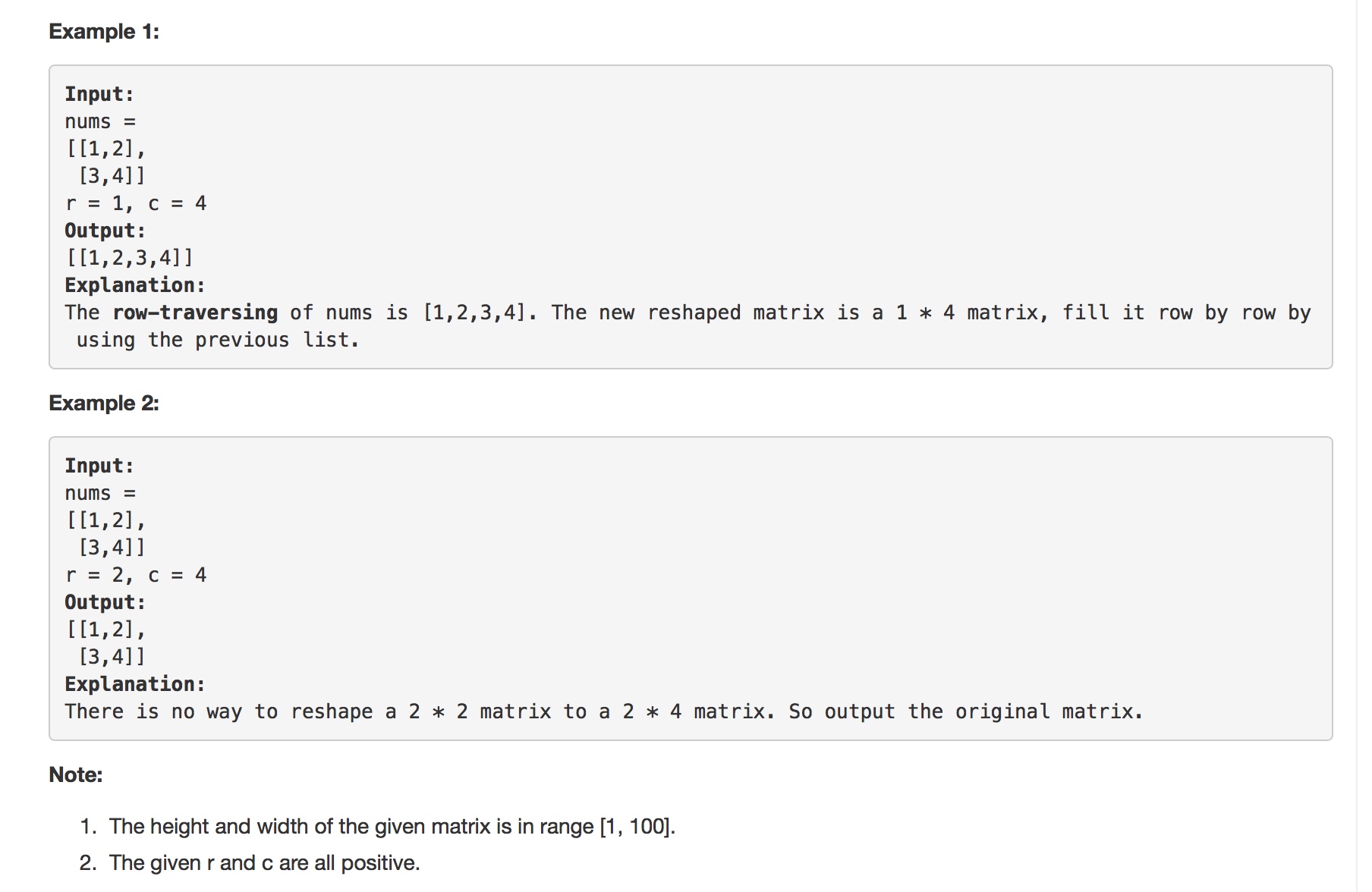
class Solution {
public:
vector<vector<int>> matrixReshape(vector<vector<int>>& nums, int r, int c) {
int sumNum = nums.size() * nums[0].size();
int r1 = nums.size();
int c1 = nums[0].size();
if (sumNum != r*c) {
return nums;
}
vector<vector<int>> result = vector<vector<int>>(r,vector<int>(c,0));
int j = 0;
for (int i =0; i<r*c; i++) {
result[i/c][i%c] = nums[i/c1][i%c1];
}
return result;
}
};