Suppose you have a long flowerbed in which some of the plots are planted and some are not. However, flowers cannot be planted in adjacent plots - they would compete for water and both would die.
Given a flowerbed (represented as an array containing 0 and 1, where 0 means empty and 1 means not empty), and a number n, return if n new flowers can be planted in it without violating the no-adjacent-flowers rule.
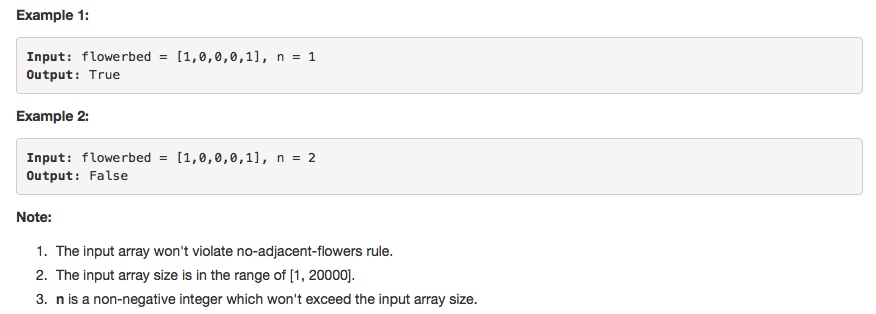
第一种:3格的滑动窗口
class Solution {
public:
bool canPlaceFlowers(vector<int>& flowerbed, int n) {
int str = 0 ,end = 2;
int tol = 0;
if (flowerbed.size()==1&&flowerbed[0]==0) {
tol++;
}else if(flowerbed[0]==0&&flowerbed[1]==0) {
flowerbed[0]=1;
tol++;
}
while (end<=flowerbed.size()-1) {
if (!(flowerbed[str]||flowerbed[str+1]||flowerbed[end])) {
flowerbed[str+1] = 1;
tol++;
}
str++;
end++;
}
if (flowerbed.size()>=3&&flowerbed[flowerbed.size()-1]==0&&flowerbed[flowerbed.size()-2]==0) {
tol++;
}
return tol>=n?1:0;
}
};
第二种:
class Solution {
public:
bool canPlaceFlowers(vector<int>& flowerbed, int n) {
for (int i = 0; i < flowerbed.size(); i++) {
if (!flowerbed[i] && (i == 0 || !flowerbed[i - 1]) && (i == flowerbed.size() - 1 || !flowerbed[i + 1])) {
flowerbed[i] = 1;
n--;
}
}
return n <= 0;
}
};